I tried using Advent of Code to learn TypeScript and it worked very well. I’m much more used to Python and often know what I’d write in that language, so asking “How do I do that in TypeScript?” for some fairly unusual topics has prompted me to learn more about this new language.
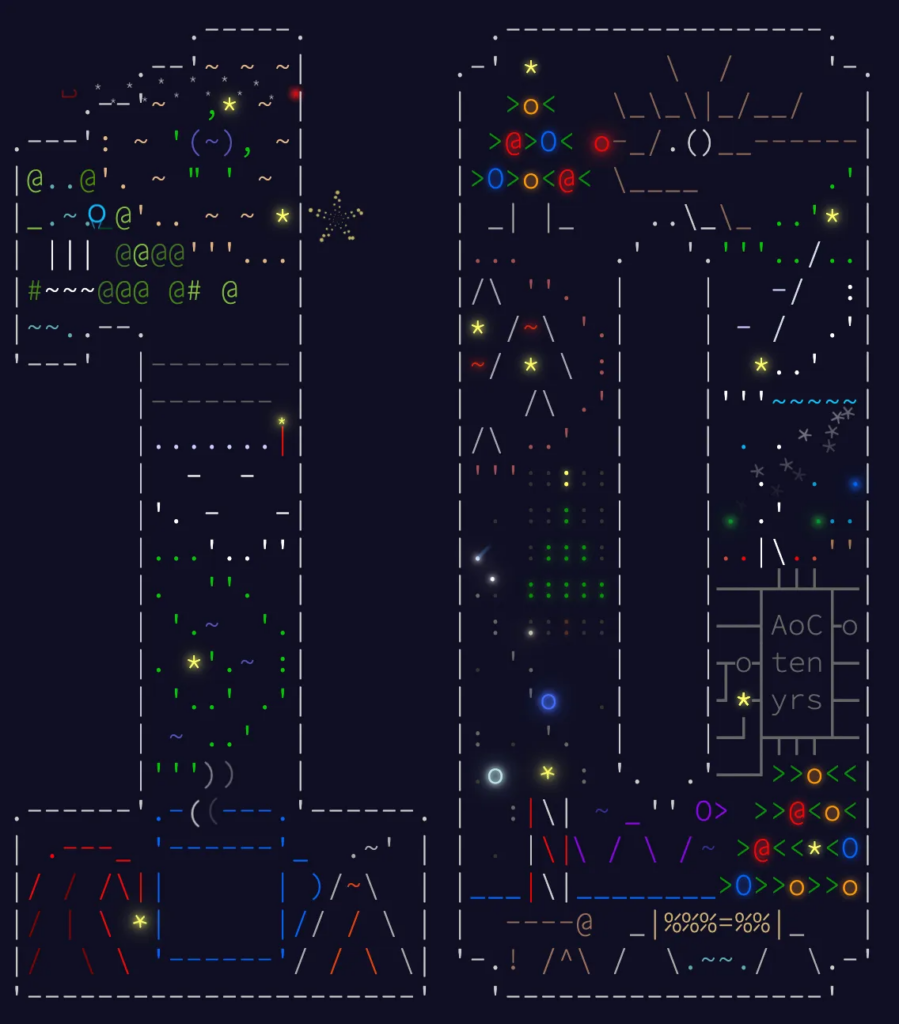
I’ve written already about memoization with decorators and generator expressions – both fun learning exercises that I was happy with the outcomes of. As always: don’t take ideas from me, a TS novice who’s twisting it to look like Python, and inflict them on your team’s production code … ask someone who knows more!
I do have a few topics I’m still stuck on – things I was very happy with in Python that I can’t see a good TypeScript / JavaScript equivalent of. If you have any suggestions for these, I’d appreciate hearing them.
I’m looking for:
- A way to use tuples as keys in sets or maps
- Profiling tools
- Anything like NumPy
Tuples as keys
In Python, tuples are value types so you can use them as map key or in sets. So if you want to store x, y coordinates and check “have I seen this one before”, you can use (0,1)
, pop lots of them in a set, get them out and use them to look up the grid. You can make a new (0,1)
and it will “equal” the old one.
In JavaScript, [0,1]
or {x:0, y:1}
are object types – so set.has({x:0, y:1})
will say “false” because it doesn’t check the x and y values, it checks whether this exact object is in there.
I can work around this by storing them as strings:
mySet.add(JSON.stringify({x:0, y:1}))
Comparisons work if you also stringify anything you want to check, and you can get the objects back with JSON.parse
.
This works, but it’s more code and conversions than I’d like. Is there anything else you’d recommend?
A colleague pointed out a proposal to add records and tuples to the language – that’s exactly what I’m after! But I’ve been disappointed before when waiting for promising-looking proposals. In 2023, when I wrote a class to do generator expressions in TypeScript, I saw there were proposals in progress to add exactly what I wanted to the language and assumed my workaround would be obsolete soon… now 2 years later those aren’t available yet.
I do appreciate that changing such a widely-used language needs careful thought, it’s been a good learning experience to see how things are decided. Similarly, picking through the 10+ year history of decorators and how versions of proposals-in-progress got implemented by various frameworks was eye opening. And I have seen helpful Set features like intersection and difference go from discussion to available in Node in just the short time I’ve been using it.
Profiling
Another “In Python I can…” topic: in Python, I was pleased with tools like cProfile to see where total cumulative time is spent, and tqdm for live-updating progress bars. These really help understand where I can improve things (e.g. is all that JSON stringifying and parsing taking much time?)
In TypeScript on Node I’ve tried the built-in node --prof
command and the 0x package, but I’m either not using them well or they’re not really suited to this kind of job. I think most Node users are more interested in time to serve up pages and respond to requests rather than CPU intensive tasks – am I right?
To show what kind of profiling I’m interested in: For one of the challenges, I wrote a first draft with lots of inefficient operations. For example, to check “have we seen this position before”, I noted all the positions it visited in an array and kept using this:
myArray.filter(g => g.x === x && g.y === y).length > 0
That was getting called millions of times, and the solution took 120 seconds to run. Changing from an array to a set cut the time down to 9 seconds:
mySet.has(JSON.stringify({x,y})
But I couldn’t get any of the profiling tools to point me to “I’m spending 90% of my time filtering an array”. It’s not always obvious where bottlenecks are – please suggest any tools that would help.
NumPy
NumPy is a library with a wide range of mathematical operations. One feature that’s very useful (in real life applications as well as some Christmas-themed coding puzzles) is its handling of multidimensional arrays: operations on these can be amazingly fast and readable. The introduction and examples describe how you can avoid nested for loops in lots of cases and specify things at a higher level, and how this lets the library take advantage of optimized C code to run much faster.
I would imagine there’s no equivalent tricks a TypeScript library could do… but maybe I’m wrong! Having a look around, I did find several packages that give nice maths operations, including some array operations that look similar to NumPy, but nothing at all that does the kind of speed up I admire in that Python library.
Suggestions appreciated
In the comments here, or on Bluesky, Mastodon or LinkedIn. Thank you!